Monday, December 19, 2005
DirectXmas
http://msdn.microsoft.com/coding4fun/
C# Static Classes
{
// Keep class from being created
private Environment() { }
}
can now be written as: public static sealed class Environment
{
}
The benefit of using a static class instead of the design pattern above is that the compiler can now report an error if any instance methods are accidentally declared.
(from link)
Sunday, December 18, 2005
C# SSH/SFTP Libraries
http://www.tamirgal.com/home/dev.aspx?Item=sharpSsh (free, but perhaps not open source)
http://www.jscape.com/articles/sftp_using_csharp.html ($299)
http://www.eldos.com/sbb/desc-sftp2.php ($559.00)
http://www.sshtools.com/showMaverickDotNet.do ($530.00)
SSH
http://www.routrek.co.jp/en/product/varaterm/granados.html (opensource)
http://www.nsoftware.com/ipworks/ssh/ ($449)
http://www.weonlydo.com/index.asp?showform=SSH.NET ($199)
updated: Thursday, Jan 12, 2006
Saturday, December 17, 2005
C# Using Statement
The using statement allows the programmer to specify when objects that use resources should release them. The object provided to the using statement must implement the IDisposable interface. This interface provides the Dispose method, which should release the object's resources.
(from MSDN VS20005 documentation)
Windows ISO Mounting Tool
Wednesday, December 14, 2005
Erasures in Java 1.5
..., the generics implementation uses a technique known as erasure. The easiest way to understand erasure is to think of the compiler as performing two distinct tasks. First, it does type checking at compile time using all the type information it has (including the type parameters). Then it transforms the code, using a set of rules that remove all of the type parameters (e.g. all of the parameterized types are mapped to raw types), and inserts a set of casting operations. The complete list of transformations is beyond the scope of this article. However, to give you an idea of what erasure does, here are some of the rules:
- If a class doesn't use type parameters in its definition, erasure doesn't change the class definition.
- Parameterized types "drop" their type parameters.
- Every type parameter is mapped to the appropriate bound. By this, I mean: the type parameter is erased and replaced with the strongest type the compiler can reasonably assert. Thus, in the case of
, T is mapped to Object. In the case of , T is mapped to Rentable, and so on. - Casts are inserted wherever necessary, to ensure that the code compiles.
Structs vs. Classes in C#
Structs vs. Classes
Structs may seem similar to classes, but there are important differences that you should be aware of. First of all, classes are reference types and structs are value types. By using structs, you can create objects that behave like the built-in types and enjoy their benefits as well.
Heap or Stack?
When you call the New operator on a class, it will be allocated on the heap. However, when you instantiate a struct, it gets created on the stack. This will yield performance gains. Also, you will not be dealing with references to an instance of a struct as you would with classes. You will be working directly with the struct instance. Because of this, when passing a struct to a method, it's passed by value instead of as a reference.
Monday, December 12, 2005
LoudNetworks in the Hizouse

Phase 1: Design and implement (efficient) reliable communication between two PCs using a microphone and speaker. CHECK
Phase 2: Design and implement robust ad hoc routing between a set of PCs using sound as their only means of communication. CHECK
Phase 3: Design and implement (efficient, fair, etc.) resource control (that is, sound control) among a set of PCs using sound as their only means of communication. CHECK
Understanding C# Timers
There are three timer controls in Visual Studio and the .NET Framework — the server-based timer you can see on the Components tab of the Toolbox, the standard Windows-based timer you can see on the Windows Forms tab of the Toolbox, and the thread timer that is only available programmatically.
- The Windows-based timer is optimized for use in Windows Forms applications.
- The server-based timer is an update of the traditional timer that has been optimized to run in a server environment.
- The thread timer is a simple, lightweight timer that uses callback methods instead of events and is served by thread-pool threads.
There are two types of threads in Win32 architecture: UI threads, and worker threads. UI threads sit idle most of the time and wait for messages to arrive in their message loops. Once they receive a message, they handle it and then wait for the next message to arrive. Alternatively, worker threads are used to perform background processing and do not use message loops. Both the Windows timer and the server-based timer run using an Interval property. The interval of the thread timer is set in the Timer constructor. The timers are designed for different purposes, as evidenced by their handling of threads:
- The Windows timer is designed for a single-threaded environment where UI threads are used to perform processing. The accuracy of Windows timers is limited to 55 milliseconds. These traditional timers require that the user code have a UI message pump available and always operate from the same thread, or marshal the call onto another thread. For a COM component, this would be detrimental to performance.
- The server-based timer is designed for use with worker threads in a multi-threaded environment. Because they use a different architecture, server-based timers have the potential to be much more accurate than Windows timers. Server timers can move among threads to handle the raised events.
- The thread timer is useful in scenarios where messages are not pumped on the thread. For example, the Windows-based timer relies on operating-system timer support, and if you are not pumping messages on the thread, the event associated with your timer will not occur. The thread timer is more useful in this case.
Windows Form Timer
This class wraps the counters we had in Win32 based on Windows messages. Use this class only if you do not plan to develop a multithreaded application. The Windows Forms Timer component has an Interval property that specifies the number of milliseconds that pass between one timer event and the next. Unless the component is disabled, a timer continues to receive the Tick event at roughly equal intervals of time. This component is designed for a Windows Forms environment. If you need a timer that is suitable for a server environment, see Introduction to Server-Based Timers.
The Interval Property
The Interval property has a few limitations to consider when you are programming a Timer component:
- If your application or another application is making heavy demands on the system — such as long loops, intensive calculations, or drive, network, or port access — your application may not get timer events as often as the Interval property specifies.
- The interval can be between 1 and 64,767, inclusive, which means that even the longest interval will not be much longer than one minute (about 64.8 seconds).
- The interval is not guaranteed to elapse exactly on time. To ensure accuracy, the timer should check the system clock as needed, rather than try to keep track of accumulated time internally.
- The system generates 18 clock ticks per second — so even though the Interval property is measured in milliseconds, the true precision of an interval is no more than one-eighteenth of a second.
Thread safety: Any public static members of this type are thread safe. Any instance members are not guaranteed to be thread safe.
Server-Based Timers (System.Timers)
The Timer component is a server-based timer, which allows you to specify a recurring interval at which the Elapsed event is raised in your application. You can then handle this event to provide regular processing. For example, suppose you have a critical server that must be kept running 24 hours a day, 7 days a week. You could create a service that uses a Timer to periodically check the server and ensure that the system is up and running. If the system is not responding, the service could attempt to restart the server or notify an administrator. The server-based Timer is designed for use with worker threads in a multithreaded environment.
Server timers can move among threads to handle the raised Elapsed event, resulting in more accuracy than Windows timers in raising the event on time. For more information on server-based timers, see Introduction to Server-Based Timers.
Key Programming Elements of Server-Based Timers
The Timer component raises an event called Elapsed. You can create handlers for this event to perform whatever processing needs to occur. Some of the more important properties and methods of a Timer component include the following:
- The Interval property is used to set the time span, in milliseconds, at which events should be raised. For example, an interval of 1000 will raise an event once a second.
- The AutoReset property determines whether the timer continues raising events after the given interval elapses. If set to true, the timer continues to recount the interval and raise events. If false, it raises one event after the interval elapses and then stops.
- The Start method sets the timer's Enabled property to true, which allows the timer to begin raising events. If the timer is already enabled, calling the Start method resets the timer.
- The Stop method sets the timer's Enabled property to false, preventing the timer from raising any more events.
Thread safety: any public static members of this type are safe for multithreaded operations. Any instance members are not guaranteed to be thread safe.
Thread-timer (System.Threading.Timer)
Use a TimerCallback delegate to specify the method you want the Timer to execute. The timer delegate is specified when the timer is constructed, and cannot be changed. The method does not execute on the thread that created the timer; it executes on a ThreadPool thread supplied by the system. The callback method executed by the timer should be reentrant, because it is called on ThreadPool threads. The callback can be executed simultaneously on two thread pool threads if the timer interval is less than the time required to execute the callback, or if all thread pool threads are in use and the callback is queued multiple times.
When you create a timer, you can specify an amount of time to wait before the first execution of the method (due time), and an amount of time to wait between subsequent executions (period). You can change these values, or disable the timer, using the Change method.
As long as you are using a Timer, you must keep a reference to it. As with any managed object, a Timer is subject to garbage collection when there are no references to it. The fact that a Timer is still active does not prevent it from being collected.
Note: System.Threading.Timer is a simple, lightweight timer that uses callback methods and is served by threadpool threads. You might also consider System.Windows.Forms.Timer for use with Windows forms, and System.Timers.Timer for server-based timer functionality. These timers use events and have additional features.
Thread safety: This type is safe for multithreaded operations.
Other Timers
1. timeGetTime() : This Windows.DLL call can give you up to 1 microsecond of accuracy on some operating systems; however it can be up to 5 microseconds on Windows NT.
2. System.Tickcount (Environment.TickCount): Gets the number of milliseconds elapsed since the system started.
3. DateTime.Ticks: The value of this property represents the number of 100-nanosecond intervals that have elapsed since 12:00:00 midnight, January 1, 0001.
4. QueryPerformanceCounter: often used in C++ Windows games. Provides a high resolution timer of less than 1 microsecond. Not exposed in managed code, so you would have to write a wrapper for it.
References
Programming the Thread Pool in the .NET Framework
System.Timers Namespace
Introduction to Server-Based Timers
Timer Programming Architecture
Introduction to the Windows Forms Timer Component
Other C# Timer Resources
Comparing the Timer Classes in the .NET Framework Class Library (added 12/15/05 5:37PM)
http://www.mvps.org/directx/articles/selecting_timer_functions.htm (added 12/20/05 2:20)
The mvps link is very revealing; it discusses timers in the context of high performance games and evaluates them via benchmarking.
Sunday, December 11, 2005
Redick vs. Morrison
J. J. Redick of Duke also had an amazing performance yesterday, scoring a career-high 41 points vs. the #2 team in the country (Texas). He shot 9/16 from the three-point line.
Katz compares the two on espn for a preliminary investigation of NCAA player of the year.
(link)
Friday, December 09, 2005
Nash a Professional Soccer Player
"'How good are you?', I asked him after his team won. 'I guess I could have played professionally,' he said. 'If I’d focused on it. Sometimes I wish I had. But that’s probably just a grass-is-always-greener kind of thing.' " [Steve Nash talking about playing soccer professionally]
(from link)
Sunday, December 04, 2005
CFP: mobilehci
Human-Computer Interaction with Mobile Devices and Services
Dates: 12-15 September, 2006
Location: Espoo, Finland
Website: www.mobilehci.org
Deadlines:
* Papers, Workshops and Tutorials: 1 March 2006
* Short Papers, Posters, Demos, Panels, Industry Cases: 7 May 2006
Saturday, November 26, 2005
Thanksgiving 2005 in Nebraska
My Mother, the Sweater Model

Tuesday, November 22, 2005
Ah, Minnesota Pride
And
"Another day, another lesson for Kris Humphries. The Jazz's second-year forward checked into the game at the start of the second quarter Saturday against Memphis. So he was obviously shocked when Robert Whaley subbed in for him just 2 1/2 minutes later. As Humphries approached the bench, Jazz coach Jerry Sloan stopped him with a blunt message. "I can't put you out there," Sloan said as Humphries passed by, "if you're not going to compete." Salt Lake Tribune
(from link)
Wednesday, November 16, 2005
College Graduation Rates
Just 54 percent of students entering four-year colleges in 1997 had a degree six years later -- and even fewer Hispanics and blacks did, according to some of the latest government figures. After borrowing for school but failing to graduate, many of those students may be worse off than if they had never attended college at all.
(from link)
Monday, November 07, 2005
Informal Awareness in a Hallway
Tuesday, November 01, 2005
What the Public Deserves
"In my administration, we will ask not only what is legal but what is right, not just what the lawyers allow but what the public deserves," Bush said Oct. 26, 2000.
(link)
Sunday, October 30, 2005
Sunday Lament
Tuesday, October 25, 2005
Fakes Words in Your Dictionary
This piece from last August's New Yorker documents the fascinating hunt for the fake word inserted into the New Oxford American Dictionary. These fake words are inserted by dictionary editors as a kind of watermark to catch competitors who copy their dictionaries wholesale. The process of figuring out which of the words in the NOAD was the fake was quite involved, with six candidates sent around to a panel of distinguished language-scholars who had a vigorous debate about whether each word was a fake
I believe that phone companies used to do something like this with their phone indexes. They would insert fake names and business (complete with phone numbers and addresses) to catch unsuspecting plagirists who would just copy the book wholesale.
Friday, October 21, 2005
Mind Blowing
Give it a try -- it really worked for me (both perceiving the green dot and the disappearance of all the pink dots).
Sunday, October 16, 2005
Gloria Mark, Mary Czerwinski, Eric Horvitz, Linda Stone
The article, titled Meet the Life Hackers, begins with highlighting Mark's research with Victor Gonzalez on how human's "multitask" in the workplace.
http://www.nytimes.com/2005/10/16/magazine/16guru.html
Tuesday, October 11, 2005
Apple in New Yorker
-Sasha Frere-Jones in the 10/10/05 New Yorker on Fiona Apple's new album
Monday, October 03, 2005
The Sky Really is Falling
An article written about security in IT cites Ed Lazowska, a faculty member in my department at UW, and begins:
Ed Lazowska, cochairman of the President’s Information Technology Advisory Committee, says that there is a looming security crisis, and the government, vendors and CIOs aren’t doing enough to stop it.
How Dare You Compare Me To Cheney
Ack. There's something fundamentally suspicious about heading a search committee that ends up choosing you. Also note to self, attempt not to follow in Cheney's footsteps in anyway such that comparisons like these could be made.
Sunday, October 02, 2005
Tuesday, September 20, 2005
Absolute, Relative, Mixed Formulas in Excel
(from link)
Friday, September 16, 2005
Mayer forms John Mayer Trio
The John Mayer Trio represents a different, harder-edged sound for the singer, songwriter and guitarist. Those fortunate enough to have tickets to the sold-out Fillmore gigs will witness a different side to the pop tunesmith known for slick hits such as ``Your Body Is a Wonderland.''
``I wouldn't just change just for the sake of changing, but I've always had another agenda,'' Mayer explains. ``That other agenda is a whole different set of heroes and hues and colors and textures. I've just decided to go to my next place. . . .
``It's a very thought-out process of taking what excites me about Eric Clapton and B.B. King and Buddy Guy and Blind Faith and Derek & the Dominos and the Band and Cream . . . and placing it in my thing. I love pop music. I love guitar playing. I love blues. I love jazz. And I love the idea even more that I could put my own stamp on all those things and roll them into one sound.''
...
``He's really serious about his guitar playing,'' Palladino says from his home in London. ``Some guitarists have a special touch, and you know the guys I'm talking about, and John is one of those guys. It's the way he strikes a note. He just has something.''
The same could be said for both Jordan and Palladino, two stellar players who have performed with rock legends.
(from link)
Monday, September 12, 2005
Well, at least we have sports...
Moment of Relief
Two weeks of suffering. A city, and region, in mourning. The mayor's pregame plea. Did New Orleans really need the Saints' 23-20 win Sunday? Len Pasquarelli says yes.
I have to remind myself why I check this page everyday? It certainly can't be for the insightful and worldly perspective.
Wednesday, September 07, 2005
Tuesday, August 23, 2005
Incredible Idea
http://www.engadget.com/entry/1234000430055701/
Cool Discovery Photos
Saturday, August 20, 2005
More Adobe Premiere Problems
I loaded the .prproj file tonight to give my friend Scott a short tutorial on Adobe Premiere. I was showing him the ins and outs of cross fades and video transitions when I noticed that one of the primary video source files was missing! Ack, what happened? I had not changed this project (or any of its dependencies) since archiving it months ago. What's the problem?
Ironically (long story) I received the lovely "red screen of death" faced me which said "media missing!." I double checked to make sure the video file was in the same place in my file system. I then tried linking, then unlinking the media through Premiere's pop-up menu. Nothing worked. Finally, I found a link on Adobe Premiere's "Support Knowledge" base which said to,
Rename the .AVI file to use the .MPG extension (for example, rename movie.avi to movie.mpg), and then import it into Adobe Premiere or Adobe Premiere Pro.
I did this and sure enough, the problem was resolved. Now what I don't understand is what caused this error? Why now? I hadn't done a thing between the last time I had used this .prprof file. Ah, yet another sad revelation of how bad Adobe Premiere really is.
Sunday, August 14, 2005
Sky Diving Accident
Friday, August 12, 2005
Sunday, July 31, 2005
Intel's Placelab in the news...
Two recent articles about PlaceLab. They both specifically mention my research :)
1. The Future of Mobile Computing
2. Intel experiments with Wi-Fi as GPS substitute.
Tuesday, July 26, 2005
You take your car to work
All along the undertows, strengthening its hold.
I never thought it would come to this, now I can never go home.
Best part is when they break it down boys choir style 2 minutes in and then proceed to rock out.
Ack, I need to stop listening to the same music I listened to in high school.
My name is Steve Vaught and I walk
http://www.thefatmanwalking.com/
Monday, July 25, 2005
K's Choice, More than an Addict
They are now an official member of my "purchased music" playlist in iTunes.
Sunday, July 24, 2005
Hot or Not Google Maps Integration
http://apps.hotornot.com/jeff/
Can't wait until they add this to:
http://www.meowmix.com/playtime/hotnot.asp
Saturday, July 16, 2005
Eclipse Ruins Then Saves
Imagine, if you will, the following frightening developer horror story:
I open Eclipse. I select some dirs to check-in. I try to commit them but I get an error. I look into a folder and think perhaps the cause is an object folder that has been bothersome (and should never be in CVS anyway). I think I select such a folder but really I select a folder containing new source code. I press delete and reflexively hit the enter button on the delete confirmation dialog.
I notice my error and panic, but only slightly. I search on Google for recovery software. I download two such tools PC Inspector File Recovery, which seemed OK but didn't find my newly deleted C# code and Restoration, which really blew and didn't find my source code files either.
Ack, now I'm getting more panicky.
I remember that Eclipse saves a local history of files. I wonder to self if Eclipse could be written such that, upon file/folder deletion through its interface, it would do one last local history backup. To calm my expectations, I figure that, nah that's probably not possible particularly when I only open the Eclipse IDE momentarily to checkin my files and not as a development environment.
I was wrong.
Eclipse saves the day. I open the local history browser and wahlah, my files! Again, stay calm. I make sure that these files are, in fact, the newly modified files and not some old, worthless version... ah, I see a new method, I see two new methods, I see all my new methods.*
Relief!
*I am a big geek.
Wednesday, July 13, 2005
XML Newbie
This link from XML.com explains it all.
Sunday, July 10, 2005
Prescription for a Queasy Stomach
Here is a listing of health inspection reports for restaurants on University Ave. in the University District at UW (note that the listing spans multiple pages). Be forewarned, however, that such listing could impact your desire to eat on the Ave. ever again. Below, I will review some of my favorite restaurants on the Ave. and their records of inspection.
But first, a briefing on the way these inspection reports supposedly work.
Inspections Overview
Inspections are based on regulations to eliminate risk factors for food borne disease. Every violation of these regulations is color coded and has a numerical value based on the amount of risk they create.
The red violations are the highest risk and pertain to things that cause food borne diseas, and the blue violations relate to maintenance and cleanliness of the restaurant. Most importantly, there is a scoring system (note that the lower the score the better).
- 35 or more red critical violation points require a re-inspection within 14 days.
- 75 or more red critical violation points require the establishment be closed.
- 101 or more total (red & blue) points also requires the establishment be closed.
Those restaurants that are forced closed are allowed to reopen when they "have remedied the conditions that have led to closure" -- Whew, that's a relief!
Interesting Finds
What I've noticed from my brief investigations is that American food places tend to do very well (e.g. Schultzy's, Big Time Brewery, even Jack in the Box), each maintaing a near perfect health inspection report (no score over 10). Schultzy's is one of my favorite places on the Ave. so I am fairly relieved there and many of my fellow Grad students hang out/eat at Big Time Brewery. Cheers to them as well.
The most common citation among all restaurants was "Food worker cards not available or current," which presumably means that (a) the restaurant is not keeping good records of their employees or (b) restaurants are hiring illegal immigrants. Admittedly (b) would be more likely in California.
Disgusting Finds (e.g. Point Winners)
- Honeybee's Cafe back in February '04 was cited with "Ineffective measures to control pests," a 13 point penalty (apparently). I'm not sure what this means exactly, but it cannot be good. It sort of has this implication that there is a pest problem to control.
- Ichiro Teriyaki scored a resounding 49 points on their last routine inspection. Cited for, among other things, improper refrigeration of food, poor handwashing facilities, unclean food contact surfaces, and, our favorite, ineffective measures to control pests.
- Never one to be out done, Aladdin Gyro-cery & Deli one-upped Ichiro Teriyaki by 7 points, scoring 56 points in 02/11/2004 for inadequate toilet facilities, unsanitary wiping clothes, improper refrigeration, inaccurate thermometers in refrigerators (the latter point here perhaps the cause of the former?). Of course, Aladdin really didn't learn much from that inspection given that one year later (02/10/2005), the restaurant scored 46 points. I hate to say it, but anyone who has eaten at Aladdin's (I have multiple times) probably isn't surprised by such a record. I'm not being perfidious, just honest.
- China Express has scored above 37 three times (see Worst Restaurants Section)
- Batavia just opened on the Ave and already received a 48 (see Worst Restaurants Section).
- Tokyo Garden. One of the few places mentioned in this blog post that I have never eaten at and perhaps for good reason. Tokyo Garden received a score of 58 points on 02/18/2005 for not storing utensils properly, poor handwashing facilities, food storage.
My all time favorite restaurant on the Ave is actually on Brooklyn Ave. Cedars offers a fantastic selection of Indian and Mediterranean food -- a seemingly strange mix, but works quite well. I really like Indian food and this is the place to do it in the U. District. The only bad part about Cedars is the table wait on weekend evenings... and their apparent "presence of pests" which are "not controlled." Cedars consistently scores in the 10-20 range in their health inspection report, most recently because of said pest issue and because of separation of living quarters/employee area, inaccurate refrigerator thermometers, etc.
My two favorite Mexican places on the Ave are, Pepes and Chipotle (yes, I know it's owned by McDonalds, shhhhh I like to eat corporate food sometimes, ok?), both of which have flawless health inspection reports never scoring even one point.
Thai food on the Ave. is fairly popular only second in prominence to Teriyaki places. I like, in order of preference: Thai 65 (just opened in early 2005), Thai Tom, Thai Spice, Thaiger Room. Overall, I am not a huge fan of Thai food (it tends to be too sweet and I don't like peanut sauce) but it's hard to avoid on the Ave.
IMO, Worst Restaurants on the Ave. (and how they fared)
The worst restaurant on the Ave is a relatively new addition to the U. District and goes by the name Batavia, which tries to pass as an authentic Indonesian restaurant. Now, I've never been to Indonesia but I hope for their sake that Batavia's version of authentic is a bit skewed. The portions are incredibly small but the food is so bad, that's actually a blessing. I went there one time and that was more than enough. Batavia received a score of a 48, which is, imo, 27 points too small. It would seem that there is some people who disagree with me on Citysearch. My guess would be, however, that those reviews were written by the owners themselves (or their kin).
The second worst restaurant on the Ave goes to Hawaii BBQ Restaurant, which managed to give myself and two others digestion problems for an entire afternoon. I went to Hawaii BBQ for the benefit of a friend who wanted to try it out and when I saw the pictures of their food on the menu posted by the door, I had difficulty staying. But I did and soon regreted it. I'm not sure what Hawaii BBQ is supposed to mean but they appeared to only have three or four authentic Hawaiin dishes -- the rest was your standard Teriyaki offering found at a dime a dozen on the Ave. But I digress, they scored a 39 at their worst inspection and an 18 (most recent) at their best.
The third worst restaurant on the Ave goes to China First, which offers a $3.50 lunch making it almost worth it suffering through their cuisine. The food here is standard Americanized Chinese food, not necessarily bad but just not good. China First has quite the health inspection report though receiving 50, 43, 38 respectively for such things as: food stored on floor, floors not properly cleaned (maybe because there was food in the way?), room temperature storage dangerous (at highest bacteria breeding temperature)
Conclusion
I feel a slight sense of guilt actually typing up these health inspection reports and publishing them on my blog. I realize that many of the restaurants on the Ave are owned and operated by families and that they are doing the best they can. It is a difficult environment, lot's of competition and little pricing flexibility. An overwhelming portion of patrons are students and, therefore, there is only so many foods and preparations that can be offered at the $6-10 range.
Fremont Outdoor Movies
My apologies to the geeks I've offended everywhere. This is yet more mounting evidence that I do not have what it takes to be a true computer scientist (dare I admit to not liking Star Trek or seeing any of those movies).
I will say, though, that I thoroughly enjoy the idea of outdoor movies. I wish that it were in a park in Fremont (perhaps a grassy knoll) rather than a parking lot. But I suppose we can't have everything -- especially when the price of admission is a suggested donation (ala The Art Institute of Chicago Museum)
* I cannot believe that Monty Python and the Holy Grail received 8.4/10 votes, beating out Braveheart, The Deer Hunter, Sixth Sense, The Graduate, Saving Private Ryan, Hotel Rwanda, The Sting, and even the Wizard of Oz (to name a few). That is blashemy and a testament of the influence that geeks have on imdb.com.
Thursday, July 07, 2005
Update: Australian Calling Rates
Well, I tried SkypeOut as well as another calling card company called Pincity, which came highly recommended to me from a friend who uses it to call Asia.
Although SkypeOut offers the cheapest calling rates of any other technology I looked into (other than computer-to-computer of course), my experience with SkypeOut was absolutely horrible. SkypeOut from Seattle, WA to an Australian cell phone costs 2 cents a minute but it is hardly worth it (rates). The audio quality was bad but the delay (over 5 seconds) was worse -- paired together, SkypeOut to Australia was unusuable. *Given that SkypeOut is essentially Voice over IP, I imagine that they will improve.
My Sprint International Calling Card that I got from Sam's club was out of minutes, so rather than recharging it I decided to try Pincity. Pincity offers incredible global calling rates to nearly everywhere in the world (rates). Calling an Australian land line costs an incredibly low $0.05 a minute; however, calling an Australian cell phone is nearly 5 times as much ($0.23 a minute). Nonetheless, I opted to try out Pincity and purchased a 10 dollar card online. Unfortunately, not only is the Australian mobile phone rate twice as high as Sprint's, the sound quality was diminished by that digitized feedback and a 2 second delay.
As soon as my Pincity account runs out (I have roughly 35 minutes left), I'm back to Sprint.
* If you're curious, SkypeOut is able to provide insanely low call rates because it uses the internet to carry most of the call to its destination and then there is only a local charge to make the call to a regular phone (link).
Tuesday, July 05, 2005
Independence Day @ Gasworks
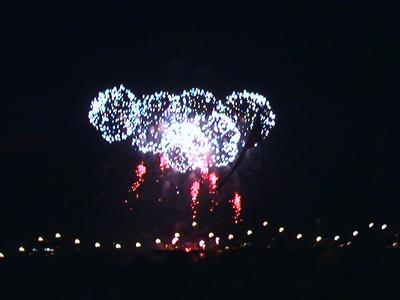
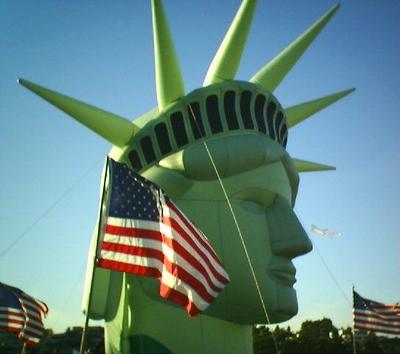
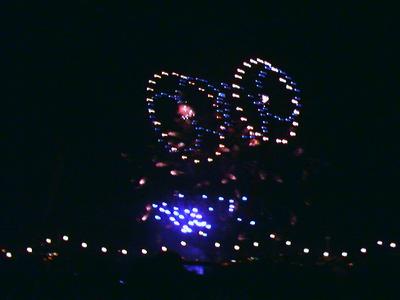
Monday, July 04, 2005
You Have a Right to Scream
Sunday, July 03, 2005
Canon S200 on Last Legs?
Which fortunately is just a picture of my Dad and not, say, an expose of Brad kissing Angelina... but you get the idea. For like a week, every picture I took with my S200 looked basically like this. Here's another one of my cousins and I at at my grandfather's house.
In some ways, I can understand why my camera would be a bit tired -- 11,173 is a lot of pictures. BEyond that it has survived two falls off a countertop onto the floor, being carried for 2 years in the front pocket of my backpack, camping trips, etc. Overall, it's been a wonderful camera - I can't really criticize it - definitely one of the best pieces of technology I've ever owned (robust, easy to use, and typically did exactly what I wanted when I wanted).
Saturday, July 02, 2005
Candy Ambivalence
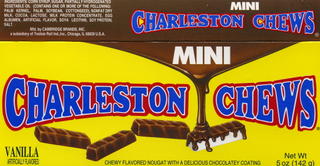
Data Loss Paranoia
Ideally my backup solution would be at some offsite location so that in the case of a fire or robbery, I would still have my data. Since I'm not a business or even a working professional, I don't have the cash to purchase a backup service over the internet. However, I'm thinking I might be able to do this on my own. Right now I'm looking at the following external USB hard drives on amazon.com:
1. $189.88 Maxtor OneTouch II Hard Drive 250 GB Hi-Speed USB (1.31 GB/dollar)
2. $189.99 SimpleTech SimpleDrive 250 GB External USB 2.0 Hard Drive (1.31 GB/dollar)
3. $220.99 LaCie 250 GB d2 External Hard Drive Extreme w/USB 2.0 (1.13 GB/dollar)
4. $244.99 Maxtor OneTouch II 300 GB USB 2.0 External Hard Drive (1.22 GB/dollar)
My plan right now is to purchase one of the above hard drives, attach it to my home computer and make an initial backup (which would be quite large), unattach the drive and then hook it up to my office computer at school. A twice a week backup would hopefully then only be incremental and therefore require little bandwidth to synchronize over the internet (e.g. from my home machine to school). I'm not sure, however, that the backup software that ships with any of these computers is capable of such an effort. I could probably use some 3rd party application like rsync...
Thursday, June 30, 2005
Tuesday, June 28, 2005
Australian Calling Rates
First, note that when calling Australia via a calling card the recipients phone line type is factored into the calling rate. Calling an Australian land line tends to be much cheaper than calling an Australian mobile (cellular) phone line. The type of telecom technology used for the originating phone call (e.g. the phone that places the call) has no impact.
Second, if the calling card is not specifically an international calling card it will most likely have a listed number of minutes. These minutes are usually for domestic nationwide long distance and NOT for international calls. You must check with the calling card company (as I did for the three examples below) to get exact rates to the region of the world you want to call. The domestic cards will then tell you the ratio of minutes charged to actual minutes talked. For example, calling Australia with one AT&T domestic phone card is charged at a 6:1 ratio. That is, for every one minute of talk time, six minutes are actually deducted from your bank of minutes.
Third, some calling cards round up to the minute others round to the second. Obviously the latter is preferred but watch out for connection fees, cards that round to the second often charge you a buck or two every time a phone call is successfully connected.
Three phone cards I investigated re: Australian rates
- $14.99 - 150 domestic long distance AT&T ($0.09 a minute)
From: Best Buy
Rate 1: 6:1 minutes ratio for USA to Australian cell phone ($0.60 a minute)
Rate 2: 3:1 minutes ratio for USA to Australian land line ($0.30 a minute) - $21.95 - 600 domestic long distance AT&T ($0.04 a minute)
From: Sam's Club
Rate 1: 12:1 minutes ratio for USA to Australian cell phone ($0.44 a minute)
Rate 2: 4:1 minutes ratio for USA to Australian land line ($0.15 a minute) - $19.98 - Sprint International Calling Card
From: Sam's Club
Rate 1: $0.11 a minute for USA to Australian cell phone
Rate 2: I'm not sure what the rate difference is to call an Australian land line.
I have been quite happy with the Sprint calling card. The line quality is good and there is no connection fee. By the way, the Australian country code is 61.
Sunday, June 26, 2005
VS2005 Visual C# Enhancements
Uses change tracking visualization concept similar to what I proposed in the Augur Eclipse plugin.
Saturday, June 25, 2005
Towering Pines
Sydney, Australia
Friday, June 17, 2005
Land O'Lakes
In Minnesota's defense, this same professor showed that humble Minnesota actually has upwards of 100,000 lakes. And, I suppose if you were to break it down density-wise, Minnesota would most definitely have Texas beat. However, there is little defense against coming in 7th out of 50 (yes, there are now 50 states in the union!) particularly because we lost to such winning states as Kansas and Oklahoma.
Of course, one argument is that many states are actually artificially increasing their lake count via reservoirs and other man-made bodies of water. They say this is for aesthetic or water irrigation reasons, I say it's because they want to beat Minnesota. Fat chance of that happening, we have Moose (Meese?).
(link)
Thursday, June 16, 2005
Morphing
Steps I used in SqirlzMorph:
1. Resize images in Adobe Photoshop taking careful note to line up the morphable object between all images -- this is essential. You can resize the images in SqirlzMorph too I think but it doesn't give you as much control to crop images so that the objects are in the same place across multiple images.
2. Open SqirlzMorph, drag your first two morph images into the interface. Maximize the SqirlzMorph interface.
3. Click the icon that shows six blue tiles in the toolbox on the left side of the SqirlzMorph interface. This will arrange your images to fill up the SqirlzMorph window.
4. OK, now we're ready to begin setting morph points. Morph points tell the software algorithm where one set of pixels in the first image translates to one set of pixels in the second image. The more morph points you place and the more accurate you place them, the better the morph will look. All morphing software that I found, used this same basic concept. Go back to that toolbox on the left side of the SqirlzMorph window, and select the icon with the black arrow AND the green plus. This will allow you to both lay down a morph point on the first picture and move its corresponding point on the second picture. Note that the red minus sign removes dots.
5. Set your first morph point on the first picture. Note that the corresponding morph point shows up on the second picture, move that second point to where it should be.
6. Do step 5 as many times as you would like -- usually the more, the better -- though this has some exceptions, particularly when your morph points don't follow some simple linear pattern for translation.
7. After your morph points are set. Go to the Morph menu in the File Menu. Select the type of Morph you want. I used One Way morph with the Mix - 1 morph algorithm.
8. Also in the Morph menu, set the Period. I used 500 frames per cycle (the more frames, the more fluid the morph).
9 . Now click on the avi icon in the toolbar on the top of the SqirlzMorph interface. Select a filename for your morph and your avi frame rate (i used 30 fps because that's what speed my video was using).
10. You've done it!
Other morphing software that I used had a much better interaction mechanism for placing, moving, and removing morph points. This is my one major criticism of SqirlzMorph.
Wednesday, June 15, 2005
Apple Places High Value on My Opinion

As a valued customer, your opinions are very important to us. That's why we would greatly appreciate your feedback regarding your iPod. Your participation in this survey will help us to better meet the needs of customers like you.Please click the link below to take the survey. This will take you to an external site hosted by Advanis, an independent marketing research firm we have engaged to perform this study. The information gathered will be for research purposes only, is strictly confidential, and will be used only by Apple. Your personal information and responses will not be shared with anyone.
The survey asked, among other things, the one thing I would like changed about my iPod, how many accessories I've purchased for my iPod, what kind of car I drive, what I think about being able to control my iPod through the steering wheel, the number of iPods in my household, if purchasing/using an iPod made me feel better about Apple, if purchasing/using an iPod made me more likely to buy an Apple computer, how I sync my iPod to my computer, how many songs I have on my iPod, how many songs I have on my computer, the number of songs I have purchased from the iTunes music store, the types of accessories I've purchased for my iPod, how I received my iPod, how I learned to use my iPod, the percentage of music that comes from downloading from the internet (not iTunes music store), ripping from CD, purchasing from iTunes music store, or other.
The survey probably took about 15 minutes and I flew through the questions. If you're curious, the only free form response question asked "what one thing would you change about the iPod?" and I said something like "The menu navigation to shuffle/repeat. The number of required menu traversals to toggle shuffle/repeat is quite high. What's most frustrating is when I am playing a song via a playlist and I want to start shuffle, I have to traverse back-up to the top level to set shuffle and then reverse traverse back down to where I was to the currently playing song (and playlist menu)."
Other things I would like to see:
* be able to play my iPod on many different computers without having to switch the iPod into "manually manage songs and playlists" mode. Ideally, I could play my iPod directly through iTunes on my work and school computers all the while keeping the iPod synched to my home computer. Note that the metadata would then stay up to date and consistent.
* when I play songs on my computer and the same songs on my iPod. The synchronizer should be smart enough to do an intelligent merge of the metadata (e.g. playcount, last played, ratings) and not just an overwrite.
* embed my ratings data (and perhaps other metadata) inside my music files themselves (mp3 id3 tags for instance)
* be able to stream my home computer iTunes library to one other computer at a time with login access.

Thursday, June 02, 2005
Adobe Premiere Success
I will say that despite the obvious advantages of using a professional-level movie editing program like Adobe Premiere over, say, freeware like Microsoft Movie Maker, the latter still has its place (at least on Windows boxes). Microsoft Movie Maker, for whatever reason, seems like it supports more formats than Adobe Premiere. I saw this when I was working off of DVD .VOB files converted into AVIs, I saw this when working with Canon S200 .avi movies, etc. All seemed to work flawlessly in Microsoft Movie Maker, but in Adobe Premiere, it took some tinkering.
Tuesday, May 24, 2005
Hallelujah
But you don't really care for music, do you?
Monday, May 23, 2005
But where are they?
Sunday, May 22, 2005
DVD Formats
The box says I can record a full DVD disc in approximately 7 minutes. I remember when it took approximately an hour to burn a full CD disc (back in 1998). A full single layer DVD disc is 4.7 GB -- where as a CD-R disc is 700 megs. I can burn 6.7 times the data nine times as fast.
The confusing part about purchasing a DVD burner is that there are all of these different capabilities (Memorex burn speeds are in paranthesis):
1. DVD+R (16x)
2. DVD-R (16x)
3. DVD+RW (8x)
4. DVD-RW (6x)
5. DVD+R9 (4x) <- this is the double layer So, great, looks like my drive supports just about every DVD format I've ever heard of (imagine how confusing this will be if the next generation DVD formats, e.g. blu-ray, don't get standardized). However, while I'm at Fry's I then wanted to know, which Disc format should I actually buy to work in the most DVD players (I eventually had to call a friend before buying a 50 disc package of DVD-Rs). Here's the skinny: http://www.manifest-tech.com/media_dvd/dvd_compat.htm#DVD-R/RW%20and%20DVD-RAM
I was really only interested in DVD-R vs. DVD+R, the link above says,
The DVD-R (Recordable) format is designed to fill much the same role with DVDs as CD-R does with CDs. It can be used to burn large data disks, and it is currently the most reliable media for burning DVD video to discs that can play back in set-top DVD players.
The DVD+R (Recordable) write-once format was defined more recently. DVD+R media and products are expected in Spring 2002. It appears that current DVD+RW drives unfortunately will not be able to write the new DVD+R media.
If you want to author DVDs, and need to be as compatible as possible with any DVD players that you might want to play them on, then go ahead with DVD-R. It works, and it will continue to work in the future. With the existing momentum, prices should remain stable and the format will not be obsolete tomorrow.
And luckily I purchased a 50 pack of DVD-Rs. However, I should note that the Memorex DVD Burner itself shipped with one DVD+R. So it looks like this is the format they are pushing.
Wednesday, May 18, 2005
Thoughts of the Curse Mixtape
Top songs so far (that I had not heard before):
Joshua Radin - Girlfriend in the Coma
Bloc Party - This Modern Love
Christopher O'Riley - Black Star
KGBtv - rx
Wow, two covers. Joshua's cover of the Smiths is absolutely gorgeous. He has a delicate, soft voice and I really like his interpretation of this song. O'Riley's cover of Radiohead is from an album of piano arrangements of Radiohead songs. I looked him up in iTunes music store (the album is called something like True Love Waits: Christoper O'Riley Plays Radiohead) and contains covers of Fake Plastic Trees, Motion Picture Soundtrack, Thinking About You, Karma Police, to name a few. My hope is that these renditions do not sound like glorified elevator music.
Anyone who knows my taste in music would not be surprised by my enthusiasm for the Bloc Party track. A perfectly repetitive guitar riff plays in the background entertwined with an intermittent piano riff which highlights the primary melody of the song. The verses are simple, terse phrases sung with dreary, stereoized vocals.
Tuesday, May 17, 2005
Number of the week: 8
[17:34] jonfroehlichUW: both over horizontal and vertical axes
[17:34] sled212: and orthogonally infinite
Monday, May 16, 2005
Breathalyzer Cellphones
The University of Washington in collaboration with Intel Research, Seattle has been working with multi-sensor microboards for a while (e.g. 2x1x2 device that contains a temp gauge, barometer, microphone, light detector, infrared detector, accelerometers, etc.). I wonder what contextual information could be derived from the addition of an ambient alcohol sensor? How much alcohol vapor is resident in that local pub down the street anyway?
(Link)
Sunday, May 15, 2005
Mixtape Challenge II (2005)
I have yet to listen to any of the submissions for Mixtape Challenge II, but here's a quick breakdown.
MOST POPULAR SONGS
Our Lady Peace - 4am (2 submissions: Milind and Rachel)
Zero 7 - Destiny (2 submissions: Adrien and Rachel)
Chicane - Saltwater (2 submissions: Martin and Melanie)
MOST POPULAR BANDS
The Decemberists (5 submissions)
Mike Doughty (5 submissions)
Black Eyed Peas (4 submissions)
Nickel Creek (4 submissions)
Old Crow Medicine Show (4 submissions)
Carla Bruni (3 submissions)
Interpol (3 submissions)
Kruger Brothers (3 submissions)
Modest Mouse (3 submissions)
The Postal Service (3 submissions)
Regina Spektor (3 submissions)
Tori Amos (3 submissions)
Yeah Yeah Yeahs (3 submissions)
And a crap load (14+) of artists w/2 submissions each.
This year's mixtape challenge also includes yet another hip-hop evangelist campaigning to "give hip-hop a chance." Here is an excerpt from his text file description:
I just thought I would take it upon myself to expose you people to more forms of hip-hop. The trash you hear on MTV just doesn't cut it for anyone, myself included, but too many people use this as an excuse to not listen to hip-hop at all. I've attempted (though, more than likely, failed miserably) to represent a wide range of underground hip-hop (many fused with other genres such as jazz and electronica) in an effort to deliver this fine form of art in a palatable manner.
I am sort of neutral on hip-hop. I don't dislike it as a genre but find that most of the music just doesn't jive with me. There are plenty of exceptions to this (e.g. Jay-Z, Kanye West, etc) yet these artists are arguably pop artists first and hip-hop artists second. The hip-hop music i like the most is strongly melodic filled with catchy hooks that underemphasize bass beats. I usually dislike the very organic, stripped down hip-hop sound -- that where the focus is simply the rhyme or the flow of voice -- i would just rather listen to something more compellingly musical (sounding).
Hip-hop evangelists are great though because (1) they see it as their duty to expose people to new music (not a bad objective and certainly one music snobs subscribe to in one form or another for their favorite genre of music) and (2) they either don't care or don't realize that hip-hop is the number one genre of music in America (overtaking Country on the billboard and sales charts a few years ago) - not necessarily a music form in the need of gospel. I realize in this particular case we are speaking of "underground" hip-hop and typically underground music is less accessible (almost experiemental by nature) than its pop counterparts so more power to him. I will indeed give this mix a chance and report back soon. In the meantime...
As the Perceptionists put it in Black Dialogue:
Yeah, it was written in the books of Europeans
we were savage
That our history was insignificant and minds below average
But how can one diminish the work
Of the most imitated culture on this earth
Fast foward to 2000 and now
You see it everywhere you look, speech, music, fasion and style
It's black dialogue
Friday, May 13, 2005
The Brunettes
So, fall back plan is amazon.com's music store (of course)! I search for Brunettes once again and, yep, you guessed it amazon.com barely carries the Brunettes -- I say barely carries because they only have an import album (for $29.49 no less -- but the album qualifies for free shipping, wee!). Here's the link in case you're fond of pricey import albums (i'm not).
So either the Brunettes have no stateside album releases or my two main music sources have failed me. Must I now be forced to try msn's online music store? The horror.
I will say this, in the age of the internet I think it's best to pick a name that's very unique yet easily spellable. I have trouble with the word Brunettes for some reason (is there two n's? is there two t's? are there both two n's and two t's? is there one n and two t's? etc.) Of course, it's not nearly as hard to spell as the band Desapareicidos. And no I did not have to look that word up -- this time.
Dim Future for CS Research in US?
The Computing Research Policy Blog has the CRA's press release, here are some highlights.
"The impact of IT research on enabling of innovation resonates far beyond just the IT sector," said James D. Foley, Chair of CRA and professor of computer science at Georgia Institute of Technology. "IT has played an essential - many argue the essential - role in the economic growth of the US in the past 20 years. In fact, the seeds of this economic growth are in the fundamental discoveries, most of which are pre-competitive and occur in the nation's universities and research laboratories," said Foley.
Congressional members seemed to agree that the US cannot afford to make compromises that would sacrifice our position as technology leaders/innovators.
Rep. Lincoln Davis (D-TN), the panel's ranking Democrat, agreed. "We cannot afford to squander our technological edge in a field that will only grow in importance."
(link to CRA Policy blog)
Archiving by Categories
Archiving of Posts
With Blogger, you get one choice: no archive, daily, weekly, OR monthly.
With TypePad, you can choose any of the above AND you also can archive by Category.
Winner - TypePad
Update 09/24/2006 @ 9:58AM: Blogger beta now allows archiving of posts by label/category (i.e., tagging) and by date
Wednesday, May 11, 2005
Movie Editing Frustrations
Background: friend sends me a DVD formatted for DVD players (e.g. .VOB files) rather than a data DVD (this would be analagous to the difference between a music CD-R that plays in any cd player and a data CD-r that only plays on a computer, he sent me the former). So, this means that I need to rip the DVD just to get at the data I want (the video). Of course, this video was originally in VHS format so when my friend digitized it he had the option of making a data DVD (he just chose not to, so he chose wrong). I used dvddecrypt to rip the DVD (it sounds all very illegal, but remember these are fricking home videos). DVDDecrypter ripped it to MPEG-2 and seemed to do a fine job, e.g. the MPEG-2 videos look comparable to the original DVD video (of course, since the original was VHS, that's not saying much) and the video plays back fine in Windows Media Player and Winamp.
Problem: I load these converted MPEG-2 videos into my video editing program. I notice immediately that the monitor window in Premiere plays the video fine but as soon as I add the video to the timeline view, the sequence window playback is horrible -- choppy, audio is not aligned to video, etc. I check on google about this and it seems that this is at least halfway normal. The sequence window shows a rendering of the video while the monitor window shows the original. OK, so I try a simple splice and edit on like a 30 second clip. I export it first into the default AVI format in Premiere. I load the AVI up into Windows Media Player and there are three problems: (1) video and audio are not synced -- the lag is inconsistent so it is not just a matter of moving the audio timeline to sync with the video; (2) video skips and replays portions over again (e.g. think cat sequence in original Matrix; (3) video is choppy.
At this point I think, OK, weird -- perhaps an issue with the export encoder. I setup like over 50 experiments this weekend to get to the bottom of this but to no avail. I try things like converting the MPEG-2 files into a different format before loading them into premiere (no dice), I try using 11 different encoders to export short 30 second edited sequence (no dice), I try using Adobe Media Encoders (separate file menu from normal export menu) (no dice), I try an old version of Adobe Premiere (6.0) (no dice), I try Pinnacle Studio Pro (no dice), I try Windows Movie Maker -- Aha! This seems to work. The Microsoft Paint of movie editing programs seems to work. Why is this? Argh. Frustration. However, Windows Movie Maker isn't much help because (1) it forces you to render things in .wmv format (i wonder why?) and (2) it is the Microsoft Paint of movie editing programs (e.g. no functionality!).
And only 2 weeks until deadline. Why me?
3/5s of My Clever Girlfriend
Secondly, I am massively upset at both Adobe Premiere 6.0 and Adobe Premiere Pro 1.0. They have literally wasted two consecutive weekends of mine. Can you say incredibly opaque interface coupled with extremely unresponsive movie previewing makes for frustrating times (note i have a 2.53 Ghz P4 with 1 gb of RAM). I also tried Pinnacle's Studio Pro -- of course this software could not (a) read .wmv files (b) export my MPEG2 video correctly.
Thirdly, I am trying a new brand of contacts today. My old brand is no longer manufactured.
Fourthly, I updated my links on the right sidebar. You should try Educate Me, it's edutaining!
Lastly, I find it ironic that the cliques, power structures, and other social dynamics found in high schools across America can also be found in faculty circles on campuses across America. Ask me if I know where the pompous elitists hang out who seem to take humor in the intellectual deprecation of others and I will simply point you to the sixth floor of my building. It's unfortunate that obtaining a PhD does not involve achieving any level of respectable moral or social principles (though I would settle for much less). I have another post brewing about this in my head that I will save for later. If you're lucky, that post will sound even more jaded.
Tuesday, May 10, 2005
Make Believe Drops Today
Here are a few of my favorite quotes from Rob Mitchum's review on pitchforkmedia.com. Disclaimer: I have yet to listen to this album but much of what he says could apply to Weezer's last album, Maladriot, anyway which to me was a collection of b-sides off of the Green Album trying to prove both that Rivers could play guitar and that he could write songs where the guitar solos weren't just rehashes of the chorus melody (read: exact copy). It was dismal. And, let's be honest, the Green Album wasn't that much better.
There are two distinct groups of bad albums. The more prevalent kind is the fodder that fills a critic's mailbox, bands with awkward names and laser-printed cover art that don't inspire ire so much as pity. The second group is more treacherous: Bands that yield high expectations due to past achievements, yet, for one reason or another, wipe out like "The Wide World of Sports"' agony-of-defeat skier... Sometimes an album is just awful. Make Believe is one of those albums.
Weezer have been given a lot of breaks in their second era-- both The Green Album and Maladroit were cut miles of slack despite consisting of little more than slightly above-average power-pop. The obvious reason for this lenience has to do with the mean age of rock critics, and the fact that most of these mid-20s scribes were at their absolute peak for bias-forming melodrama when The Blue Album and Pinkerton were released.
Hearing a song like "We Are All on Drugs", which nicks the classic melody of the schoolyard "Diarrhea" song (you know, "when you're sliding into first..." and so on) for an anti-drug message stiffer than Nancy Reagan's "Diff'rent Strokes" cameo, it calls into question whether The Blue Album was really that great, or whether it just stood out as a rare beacon of guitar pop in a grunge-obsessed era.
The one half-decent song on the record, "This Is Such a Pity", fails to even maintain its status as a pleasant Cars homage, interjecting a guitar solo that sounds like it was cut from the original score to Top Gun.
And the absolute best...
So does Make Believe completely ruin not just present-day Weezer, but retroactively, any enjoyment to be had from their earlier work? I don't know-- I'm too scared to re-listen to those first two albums-- but it certainly appears that Make Believe will expertly extract the last remaining good graces the critical community has to offer latter-day Weezer, unless my colleagues' memories of slow-dancing with Ashley to "Say It Ain't So" are more powerful than I can possibly imagine. Of course, if Ashley went on to break your heart, fellow critic, Make Believe might be just the medicine you need; put it on repeat and watch your emotional scar be obliterated as collateral damage in the torpedoing of Weezer's legacy.
Touche my dear critic. Touche!
Monday, May 09, 2005
Yunnie Irony
1With a growing Chinese American population, the press, such as the Orange County edition of the Los Angeles Times, have considered Irvine to be "a [suburban] Chinatown in the making" and "becoming Orange County's Chinatown". (from wikipedia)
Sunday, May 08, 2005
It's 7:37PM and...
signed,
extremely frustrated
Friday, May 06, 2005
Yahoo API vs. Google API
Yahoo! API exposes:
Google API exposes:
- Google's main web index
Note that Yahoo! has taken a much more liberal approach in opening up more facets of their search engine. Based on the following, it's easy to ascertain that Yahoo is really looking to support developers here (perhaps in hopes of motivating developers to use their services over Google). I'm not sure if MSN search has an open API yet. Some other differences I observed while comparing their FAQs are below: Google is in blue while Yahoo! is in red.
Communication Implementation
How does the Google Web APIs service work?
Developers write software programs that connect remotely to the Google Web APIs service. Communication is performed via the Simple Object Access Protocol (SOAP), an XML-based mechanism for exchanging typed information.
Q: Why is Yahoo! using REST?
Our goal is to make Yahoo! Search Web Services available to as many developers as possible. REST based services are easy to understand and accessible from most modern programming languages. In fact, you can get a fair amount done with only a browser and your favorite scripting language.
Q: Does Yahoo! plan to support SOAP?
Not at this time. We may provide SOAP interfaces in the future, if there is significant demand. We believe REST has a lower barrier to entry, is easier to use than SOAP, and is entirely sufficient for these services.
Access Authentication
How do I get access to the Google Web APIs service?
You must first agree to the terms and conditions of the Google Web APIs service and create a Google Account. Google will then email you a license key to use with your Google Web APIs applications. Every time you make a call to the Google Web APIs service, you must include your license key.
Can I create more than one Google Account to get multiple license keys?
No. The terms and conditions you agree to restrict you one account for your personal use. Users who attempt to create more than one account are subject to being banned from the Google Web APIs service.
Q: Do I need a developer token to access Yahoo! API's?
No. Yahoo! Search Web Services use an application ID, not a developer ID.We require an Application ID to be sent with each request. This ID identifies your application, not you, the developer.Application IDs are not used to limit access to the services. Access is rate limited based on the caller's IP address. Each service may have a different access rate limit, and if you exceed that rate, you won't be able to use it for a set amount of time. The documentation for each service lists its rate limit.Best of all you can distribute your great Yahoo! Web Service-powered applications to all of your friends. So long as they're accessing Yahoo! Search Web Services from a different IP, their use will not detract from your limit. If they abuse the service from their computer, your access will not be affected.
Q: What is an Application ID?
An Application ID is a string that uniquely identifies your application. Think of it as like a User-Agent string. If you have multiple applications, you should use a different ID for each one. You can register your ID and make sure nobody is already using your ID on our Application ID registration page.
Rate Limits
How many queries can I issue from my computer programs?
Google provides each developer who registers to use the Google Web APIs service a limit of 1,000 queries per day.
Why is the daily limit only 1,000 queries?
The Google Web APIs service is an experimental free program, so the resources available to support the program are limited.
What if I want to pay Google for the ability to issue more than 1,000 queries per day?
Google is only offering the free beta service at this time. If you would like to see Google develop a commercial service, let us know at api-support@google.com.
Q: What are the limits on how much I can use Yahoo! Search Web Services?
Each service may have different rate limits. See the documentation for each service for more information. (As an aside, the image search has a limit of 5,000 queries per day per ip per application ID!)
Q: How will I know when I hit a limit?
When a rate limit has been exceeded, the HTTP request will receive an error code of 403; along with a standard XML error response. See our rate limiting documentation for details.
Results Per Query
Is there a limit on the number of results I can receive per query?
Yes. You can retrieve a maximum of 10 results per query, and you cannot access information beyond the 1000th result for any given query.
Q: What is the limit on the number of search results I can receive per query?
Each service may have different limits on the number of results you can receive. The documentation for that service will specify the default number and maximum number you can receive. Most services are limited to 50 results per query. Local Search is limited to 20 results per query.
Tracking Usage
How can I track the number of queries I have submitted each day?
Developers need to perform their own tracking of their daily usage.
Q: How can I track the number of queries I have submitted?
If you're a developer, please keep track in your code. Also, consider exposing the counts to the users of your application so they'll have a way to track their usage as well.
Q: How can I track the usage of my applications?
You can get daily usage reports for each of your Application IDs.